HTML5 Games
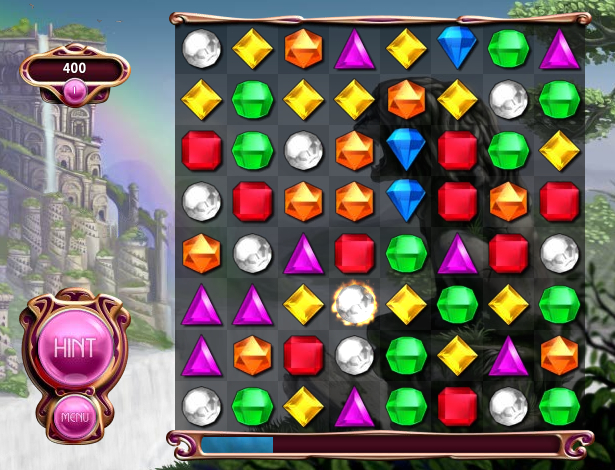
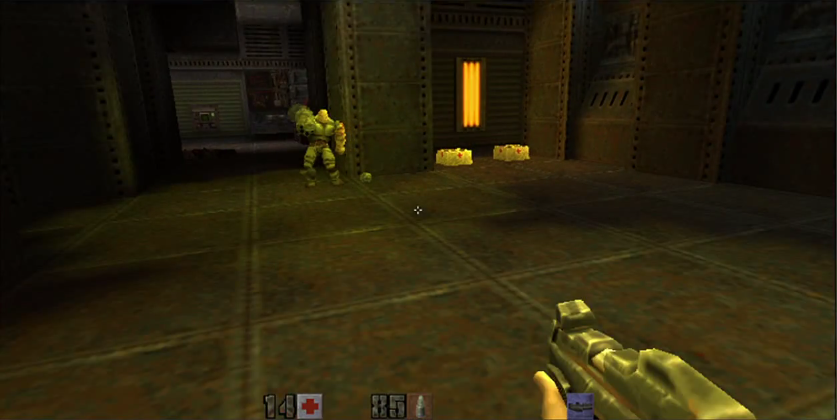
public class MyGame implements Game { public void init() { // initialize game. } public void update(float delta) { // update world: // delta indicates the time-step } public void paint(float alpha) { // render world: // alpha indicates time in the range [0, 1) between world frames } }
pointer().setListener(new Pointer.Adapter() { public void onPointerStart(Pointer.Event event) { // Handle mouse down event. } }); keyboard().setListener(new Keyboard.Adapter() { public void onKeyDown(Event event) { // Handle key down event. } });
public void init() { bg = graphics().createSurfaceLayer(); graphics.rootLayer().add(bg); Layer catGirl = graphics().createImageLayer('catGirl.png'); graphics.rootLayer().add(catGirl); } public void paint(float alpha) { Surface surf = bg.surf(); surf.clear(); surf.drawImage(cloud, cloudX, cloudY); }
public void init() { Sound music = assets().getSound('ambient.mp3'); music.setLooping(true); music.play(); squawk = assets().getSound('squawk.mp3'); } public void somethingHappened() { squawk.play(); }
public void init() { Image image = assets().getImage('bird.png'); Sound sound = assets().getSound('squawk.mp3'); // Completion callbacks are available image.addCallback(new ResourceCallback<Image>() { public void done(Image resource) { imageReady = true; } public void error(Throwable err) { imageFailed(); } }); // Text is necessarily async assets().getText('level.json', new ResourceCallback<String>() { public void done(String resource) { loadLevel(json().parse(resource)); } public void error(Throwable err) { gameOver(); } }); }
public void saveState() { Writer json = json().newWriter(); json.key('id'); json.value(playerId); json.key('score'); json.value(playerScore); net().post('/saveState', json.write(), new Callback<String>() { public void onSuccess(String result) { } public void onFailure(Throwable cause) { tryAgain();} }); }
public void init() { world = new World(gravity, true); Body ground = world.createBody(new BodyDef()); PolygonShape groundShape = new PolygonShape(); groundShape.setAsEdge(new Vec2(0, height), new Vec2(width, height)); ground.createFixture(groundShape, 0.0f); world.setContactListener(new ContactListener() { public void beginContact(Contact contact) { ... } public void endContact(Contact contact) { ... } // ... } } public void update(float delta) { // Fix physics at 30f/s for stability. world.step(0.033f, 10, 10); }
public class MyGame implements Game { public void init() { int width = 640; int height = 480; CanvasImage bgImage = graphics().createImage(width, height); Canvas canvas = bgImage.canvas(); canvas.setFillColor(0xff87ceeb); canvas.fillRect(0, 0, width, height); ImageLayer bg = graphics().createImageLayer(bgImage); graphics().rootLayer().add(bg); } }
Image cloudImage = assets().getImage("images/cloud.png"); ImageLayer cloud = graphics().createImageLayer(cloudImage); graphics().rootLayer().add(cloud); float x = 24.0f; float y = 3.0f; cloud.setTranslation(x, y);
public void paint(float delta) { x += 0.1f * delta; if (x > bgImage.width() + cloudImage.width()) { x = -cloudImage.width(); } cloud.setTranslation(x, y); }
Image ballImage = assetManager().getImage("images/ball.png"); GroupLayer ballsLayer = graphics().createGroupLayer(); graphics().rootLayer().add(ballsLayer); pointer().setListener(new Pointer.Adapter() { @Override public void onPointerEnd(Pointer.Event event) { ImageLayer ball = graphics().createImageLayer(ballImage); ball.setTranslation(event.x(), event.y()); ballsLayer.add(ball); } });
float physUnitPerScreenUnit = 1 / 26.666667f; Vec2 gravity = new Vec2(0.0f, 10.0f); world = new World(gravity, true); ballsLayer.setScale(1f / physUnitPerScreenUnit);
class Ball { public initPhysics() { BodyDef bodyDef = new BodyDef(); bodyDef.type = BodyType.DYNAMIC; body = world.createBody(bodyDef); FixtureDef fixtureDef = new FixtureDef(); fixtureDef.shape = new CircleShape(); fixtureDef.circleShape.m_radius = 0.5f; fixtureDef.density = 1.0f; body.createFixture(fixtureDef); } }
float worldWidth = physUnitPerScreenUnit * width; float worldHeight = physUnitPerScreenUnit * height; Body ground = world.createBody(new BodyDef()); PolygonShape groundShape = new PolygonShape(); groundShape.setAsEdge(new Vec2(0, worldHeight), new Vec2(worldWidth, worldHeight)); ground.createFixture(groundShape, 0.0f);
Image blockImage = assetManager().getImage("images/block.png"); float blockWidth = blockImage.width() * physUnitPerScreenUnit; float blockHeight = blockImage.height() * physUnitPerScreenUnit; GroupLayer blocksLayer = graphics().createGroupLayer(); blocksLayer.setScale(1f / physUnitPerScreenUnit); Body blocksBody = world.createBody(new BodyDef());
for (int i = 0; i < 4; i++) { ImageLayer block = graphics().createImageLayer(blockImage); block.setTranslation((1+2*i)*blockWidth, worldHeight-height); blocksLayer.add(block); PolygonShape shape = new PolygonShape(); shape.setAsBox(blockWidth/2f, blockHeight/2f, block.transform().translation(), 0f); blocksBody.createFixture(shape, 0.0f); }
public void initNails() { for (int x = 100; x < bgImage.width() - 100; x += 50) { for (int y = 150; y < 450; y+= 100) { canvas.setFillColor(0xffaaaaaa); canvas.fillCircle(x, y, radius); CircleShape circleShape = new CircleShape(); circleShape.m_radius = 5f*physUnitPerScreenUnit; circleShape.m_p.set(x*physUnitPerScreenUnit, y*physUnitPerScreenUnit); FixtureDef fixtureDef = new FixtureDef(); fixtureDef.shape = circleShape; fixtureDef.restitution = 0.6f; ground.createFixture(fixtureDef); } } }
int[] pointsTable = {-10, 10, 50, 10, -10}; int points = 0; public void update(float delta) { for (Ball ball : balls) { Vector pos = ball.layer.transform().translation(); if (pos.y() >= scoringHeight) { int slot = (int)pos.x() / (int)scoringWidth; points += pointsTable[slot]; points = Math.max(0, points); ballsLayer.remove(ball.layer); world.destroyBody(ball.body); removeBalls.add(ball); } } }
Image pointsFontImage; void init() { pointsLayer = graphics().createGroupLayer(); pointsLayer.setScale(3.0f, 3.0f); pointsFontImage = assetManager().getImage("images/font.png"); graphics().rootLayer().add(pointsLayer); } void update { float x = 0f; pointsLayer.clear(); for (char c : Integer.toString(points).toCharArray()) { ImageLayer digit = graphics().createImageLayer(pointsFontImage); digit.setSourceRect((c - '0' + 9) % 10; * 16, 0, 16, 16); pointsLayer.add(digit); digit.setTranslation(x, 0f); x += 16f; } }