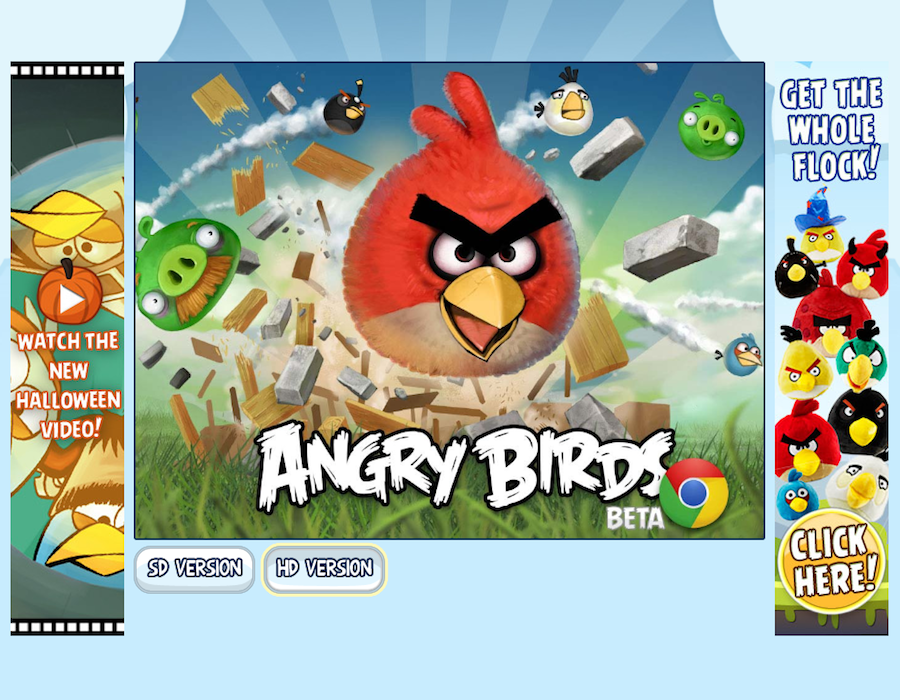
< div style=' background: url(pig.png) no-repeat; -webkit-transform: matrix3d( m00, m10, 0, tx, m01, m11, 0, ty, 0, 0, 1, 0, 0, 0, 0, 1 ); ' /> |
var ctx = canvas.getContext( '2d' ); ctx.save(); ctx.transform(m00, m01, m10, m11, tx, ty); ctx.drawImage(pigImg, 0, 0); ctx.restor(); |
var positions = new Float32Array([0, 0, 0, 1, 1, 1, 1, 0]); gl.bufferSubData(gl.ARRAY_BUFFER, 0, positions); var texCoords = new Float32Array([0, 0, 0, 1, 1, 1, 1, 0]); gl.bufferSubData(gl.ARRAY_BUFFER, 0, texCoords); gl.bindTexture(gl.TEXTURE_2D, tex); gl.texImage2D(gl.TEXTURE_2D, 0, gl.RGBA, gl.RGBA, gl.UNSIGNED_BYTE, pig); gl.vertexAttribPointer(posAttr, 3, gl.FLOAT, false , 0, 0); gl.vertexAttribPointer(texAttr, 2, gl.FLOAT, false , 0, 12 * 4); gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, indexBuffer); gl.drawArrays(gl.TRIANGLE_STRIP, 0, 4); |
function mul(A, v, out) { out.x = v.x * A.m00 + v.y * A.m10; out.y = v.x * A.m01 + v.y * A.m11; } var A = new Mat(1, 0, 0, 1); var v = new Vec(0, 0); mul(A, v, out); |
function mul(A, v, out) { out[0] = v[0] * A[0] + v[1] * A[2]; out[1] = v[0] * A[1] + v[1] * A[3]; } var A = new Float32Array(1, 0, 0, 1); var v = new Float32Array(0, 0); mul(A, v, out); |
< img src = 'bird0.png' > |
public class MyGame implements Game { public void init() { // initialize game. } public void update( float delta) { // update world: // delta indicates the time-step } public void paint( float alpha) { // render world: // alpha indicates time in the range [0, 1) between world frames } } |
pointer().setListener( new Pointer.Adapter() { public void onPointerStart(Pointer.Event event) { // Handle mouse down event. } }); keyboard().setListener( new Keyboard.Adapter() { public void onKeyDown(Event event) { // Handle key down event. } }); |
public void init() { bg = graphics().createSurfaceLayer(); graphics.rootLayer().add(bg); Layer catGirl = graphics().createImageLayer( 'catGirl.png' ); graphics.rootLayer().add(catGirl); } public void paint( float alpha) { Surface surf = bg.surf(); surf.clear(); surf.drawImage(cloud, cloudX, cloudY); } |
public void init() { Sound music = assetManager().getSound( 'ambient.mp3' ); music.setLooping( true ); music.play(); squawk = assetManager().getSound( 'squawk.mp3' ); } public void somethingHappened() { squawk.play(); } |
public void init() { Image image = assetManager().getImage( 'bird.png' ); Sound sound = assetManager().getSound( 'squawk.mp3' ); // Completion callbacks are available image.addCallback( new ResourceCallback<Image>() { public void done(Image resource) { imageReady = true ; } public void error(Throwable err) { imageFailed(); } }); // Text is necessarily async assetManager().getText( 'level.json' , new ResourceCallback<String>() { public void done(String resource) { loadLevel(json().parse(resource)); } public void error(Throwable err) { gameOver(); } }); } |
public void saveState() { Writer json = json().newWriter(); json.key( 'id' ); json.value(playerId); json.key( 'score' ); json.value(playerScore); net().post( '/saveState' , json.write(), new Callback<String>() { public void onSuccess(String result) { } public void onFailure(Throwable cause) { tryAgain();} }); } |
public void init() { world = new World(gravity, true ); Body ground = world.createBody( new BodyDef()); PolygonShape groundShape = new PolygonShape(); groundShape.setAsEdge( new Vec2( 0 , height), new Vec2(width, height)); ground.createFixture(groundShape, 0 .0f); world.setContactListener( new ContactListener() { public void beginContact(Contact contact) { ... } public void endContact(Contact contact) { ... } // ... } } public void update( float delta) { // Fix physics at 30f/s for stability. world.step( 0 .033f, 10 , 10 ); } |